Goal
- How to impletement the function which support file download in Django
Intro
- I wanted to make people download apk file in my REST server
Practice
1. Create App and setup files
1.1. Start app
(venv) $ python manage.py startapp [APP_NAME]

1.2 Setup files to be downloaded
- You can put files in anywhere, but I made ‘files’ directory in created app directory. And I put my files in there.
- In my case, I created new sub directory name ‘apk’ and put my apk files in there.
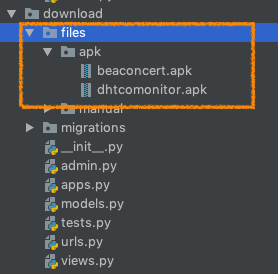
2. Create function for download
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31 | ############################################################
#
# Request for downloading
#
############################################################
# Version Date Author Description
############################################################
# 1.0.0 2019-06-26 dorbae Initialize
############################################################
from django.http import HttpResponse, Http404
import os
# Django project base directory
BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
#
# Download APK file
#
def download_apk(request, file_base_name):
print('Download ' + file_base_name + '.apk...')
# Full path of file
file_path = BASE_DIR + '/download/files/apk/' + file_base_name + '.apk'
if os.path.exists(file_path):
with open(file_path, 'rb') as fh:
response = HttpResponse(fh.read(), content_type="application/force_download")
response['Content-Disposition'] = 'inline; filename=' + os.path.basename(file_path)
return response
# If file is not exists
raise Http404
|
3. URL Mapping
3.1. Edit [APP]/urls.py
1
2
3
4
5
6
7 | from django.urls import path
from . import views
urlpatterns = [
path('apks/<str:file_base_name>/', views.download_apk, name='download_apk'),
]
|
3.2. Edit [PROJECT]/urls.py
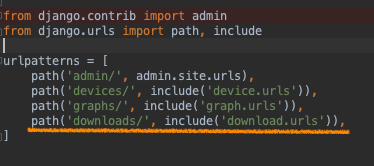
4. Test

References
댓글남기기